Mastering Currying in JavaScript
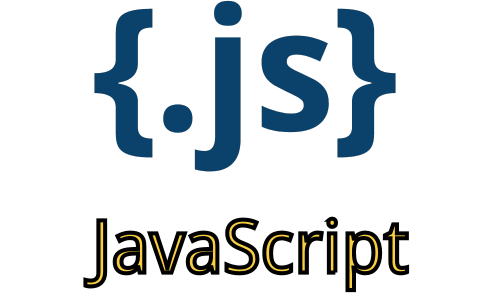
What is Currying? Currying is a functional programming technique that transforms a function with multiple arguments into a series of functions, each accepting a single argument. The name "currying" pays homage to Haskell Curry, an influential mathematician and logician.
Understanding the Benefits: To grasp the benefits of currying, let's explore its advantages in more detail:
-
Partial Function Application: Currying allows us to apply arguments to a function partially. That means we can fix some arguments in advance, creating new specialized functions ready to accept the remaining arguments. Partial function application promotes code reuse and simplifies complex computations.
-
Flexibility and Modularity: Currying enhances the flexibility and modularity of our code. We can compose them together more efficiently by breaking down functions with multiple arguments into smaller, single-argument functions. This flexibility enables us to build reusable and adaptable code blocks.
-
Function Composition: Currying is crucial in function composition, a powerful concept in functional programming. Function composition involves combining multiple functions to create a new function. Currying simplifies the process of composing functions by enabling the output of one function to be seamlessly passed as input to another function.
Example 1: Basic Currying Let's start with a basic example to illustrate the concept of currying:
Loading...
In this example, the
Loading...
function takes an argumentLoading...
a' to its argument. By partially applyingLoading...
withLoading...
, we create a new functionLoading...
. This curried function addsLoading...
to any argument passed to it.Example 2: Practical Use Case Now, let's consider a practical scenario where currying proves particularly useful. Suppose we need to calculate the total price of items based on their base price and the applicable tax rate:
Loading...
In this example, the
Loading...
function takes theLoading...
as an argument and returns a function that calculates the total price based on the providedLoading...
. By partially applyingLoading...
withLoading...
, we create a reusable functionLoading...
Subsequently, by providingLoading...
as theLoading...
, we obtain the final result ofLoading...
.Understanding the Benefits: To grasp the benefits of currying, let's explore its advantages in more detail:
-
Partial Function Application: Currying allows us to apply arguments to a function partially. That means we can fix some arguments in advance, creating new specialized functions ready to accept the remaining arguments. Partial function application promotes code reuse and simplifies complex computations.
-
Flexibility and Modularity: Currying enhances the flexibility and modularity of our code. We can compose them together more efficiently by breaking down functions with multiple arguments into smaller, single-argument functions. This flexibility enables us to build reusable and adaptable code blocks.
-
Function Composition: Currying is crucial in function composition, a powerful concept in functional programming. Function composition involves combining multiple functions to create a new function. Currying simplifies the process of composing functions by enabling the output of one function to be seamlessly passed as input to another function.
Best Practices for Currying: To make the most of currying in your JavaScript code, consider the following best practices:
-
Order of Arguments: When currying functions, it's essential to consider the order of the arguments carefully. Place the arguments that are less likely to change towards the beginning, allowing for a more accessible partial application of those arguments.
-
Currying Utility Functions: Consider creating utility functions or helper libraries to handle currying. These utilities can provide reusable currying functions easily integrated into your codebase. Popular libraries like Lodash and Ramda provide powerful currying utilities.
-
Maintain Clear Function Signatures: Ensure that curried functions have clear and descriptive names, reflecting the purpose of each partial application. That promotes code readability and makes it easier for other developers to understand and use your functions.
-
Currying and Error Handling: Handling errors effectively when currying functions is essential. Ensure that appropriate error-handling mechanisms, such as validation or exception handling, are in place to take missing or invalid arguments during partial application.
-
Avoid Over-Currying: While currying can be a powerful technique, it's essential to strike a balance and avoid over-currying. Excessive currying may lead to overly complex code and reduced readability. Consider the complexity and frequency of partial application in your specific use case to find the right balance.
Currying is a powerful technique in JavaScript that allows us to transform functions with multiple arguments into reusable, single-argument functions. By applying arguments partially, we can create specialized functions, enhance code modularity, and simplify complex computations. By following best practices, such as carefully ordering arguments, using utility functions, and maintaining clear function signatures, we can leverage the full potential of currying in our JavaScript code.
Practice Questions: To solidify your understanding of currying, here are some practice questions:
- Write a curried function that multiplies two numbers and returns the result.
- Implement a curried function to concatenate three strings together.
- Create a curried function to calculate the
** Book Recommendation: Eloquent JavaScript
Remember, if you get stuck, don't be afraid to look up solutions or ask for help. The key to learning programming is persistence! Ask for help - Mentorship
Join Our Discord Community Unleash your potential, join a vibrant community of like-minded learners, and let's shape the future of programming together. Click here to join us on Discord.
For Consulting and Mentorship, feel free to contact slavo.io