Parameters vs Arguments in JavaScript
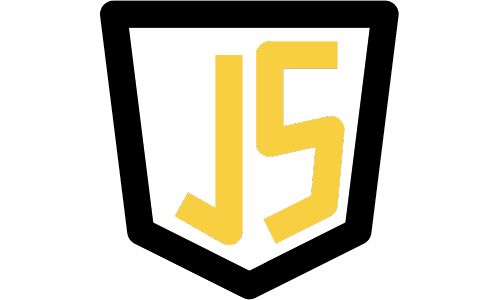
In JavaScript, "parameters" and "arguments" are often used interchangeably, but they refer to two distinct concepts in the context of functions. Understanding their differences is crucial for anyone learning JavaScript or building more complex applications.
What Are Parameters?
Parameters are essential elements in JavaScript functions. They act as placeholders for data passed to the function during its execution. They define the expected input for a function, enabling it to work with different values dynamically. This section will dive deeper into what parameters are, how they work, and their importance in JavaScript programming.
Defining Parameters
When you declare a function, parameters are defined within the parentheses following the function name. They represent the expected data that the function requires to perform its operation. The parameter names should be descriptive enough to indicate their intended use, but they are essentially variable names that will receive values when the function is called.
Here's an example of a simple function with two parameters:
Loading...
In this example,
Loading...
andLoading...
are parameters. They don't have any specific values yet—they are placeholders for the actual data that will be used when the function is called.Parameters in Action
Parameters allow a function to be reused with different inputs, making your code more flexible and modular. When you call a function, you provide the data (arguments) that replace the parameters. The function then uses those values to perform its task.
Let's consider an example where a function has three parameters to calculate the volume of a box:
Loading...
In this case,
Loading...
,Loading...
, andLoading...
are parameters. They define the expected input that the function needs to calculate the volume. When you call the function, you provide specific arguments to replace the parameters, allowing the function to perform the calculation:Loading...
The Importance of Parameters
Parameters play a crucial role in making functions reusable and adaptable. Using parameters, you can create generic functions that work with various inputs. This approach promotes code reusability and reduces redundancy, as you don't need to write separate functions for every specific case.
Consider a scenario where you must create a function that adds two numbers. Without parameters, you would need to create a new function for each specific addition:
Loading...
This approach needs to be more flexible and scalable. With parameters, you can create a single function that can add any two numbers:
Loading...
Default Parameters
JavaScript allows you to set default values for parameters, ensuring that even if an argument isn't provided during function execution, the function still has a value to work with. Default parameters help create more robust functions that handle various scenarios without causing errors.
Here's an example of a function with a default parameter:
Loading...
In this example, the parameter
Loading...
has a default value of "Guest." If no argument is provided when calling the function, the default value is used, ensuring the function always has a valid input.Parameters are a fundamental concept in JavaScript, allowing functions to be flexible, reusable, and adaptable. By defining parameters, you create a blueprint for the data that a function needs, enabling you to pass different values during function execution. This approach fosters code reusability, promotes modular design, and reduces redundancy in your code.
What Are Arguments?
In JavaScript, arguments refer to the actual values or data passed to a function when it's called. While parameters represent the expected inputs for a function, arguments are the fundamental values that take the place of those parameters during the function's execution. This section will explore arguments, their use, and their significance in JavaScript programming.
Understanding Arguments
When you call a function in JavaScript, you typically pass in one or more arguments. These arguments replace the parameters defined in the function declaration. The function uses these arguments to perform its operations, making arguments a key component of function execution.
Here's a simple example with a function that adds two numbers:
Loading...
In this example,
Loading...
andLoading...
are the parameters, whileLoading...
andLoading...
are the arguments passed when the function is called. The function then uses these arguments to compute the sum and return the result.Passing Arguments to Functions
Arguments can be passed to functions in various ways, allowing for flexibility in how functions are used. Here are some common methods for passing arguments:
-
Positional Arguments: Positional arguments are passed to functions based on their order. The first argument corresponds to the first parameter, the second argument to the second parameter, and so on.
Loading...
-
Default Arguments: Default arguments are used when a function is called with fewer than parameters. The parameters without corresponding arguments are given default values to ensure the function has valid input.
Loading...
-
Rest Arguments: Rest arguments allow a function to accept a variable number of arguments, grouping them into an array for processing. This feature is useful when the number of arguments still needs to be fixed.
Loading...
Arguments and Function Flexibility
The ability to pass different arguments to a function makes it flexible and reusable. By providing different arguments, you can create a single function and use it in multiple contexts, allowing for a more modular approach to coding.
For example, consider a function that calculates the area of a rectangle:
Loading...
This function can be used with different arguments to calculate the area of various rectangles:
Loading...
The same function can handle multiple scenarios by passing different arguments, reducing code redundancy, and promoting reusability.
Argument Validations and Errors
When passing arguments to functions, ensuring they match the expected data types and meet any required constraints is crucial. Failure to do so can result in errors or unexpected behavior. For example, calling a function with fewer arguments than expected can lead to undefined parameters, while passing arguments of the wrong type can cause type-related errors.
Here's an example where a function receives fewer arguments than expected, resulting in an error:
Loading...
This behavior can be mitigated by validating arguments or setting default values for parameters to handle missing arguments.
Arguments are a critical concept in JavaScript, representing the values passed to a function during execution. They enable flexible, adaptable, and reusable functions, allowing developers to create modular code that can handle various inputs. Understanding how arguments work, their role in function calls, and best practices for their use is essential for anyone learning or working with JavaScript.
The Relationship Between Parameters and Arguments
Parameters and arguments are interconnected in JavaScript. While parameters represent the expected input in a function's definition, arguments are the values passed to that function during its execution. The relationship between parameters and arguments drives the core functionality of functions in JavaScript, making them versatile and dynamic. This section explores the link between parameters and arguments, emphasizing their relationship's basic and advanced aspects.
Parameters Define Expectations
Parameters in JavaScript serve as placeholders in a function's definition. They establish the blueprint for the kind of data the function requires. When you define a function, you declare parameters to indicate what values the function expects when it's called. Here's an example of a function with parameters:
Loading...
In this example,
Loading...
andLoading...
are parameters. They set the expectation for the function: it needs two numbers to perform multiplication. Parameters make a function reusable because they let you call the function with different data sets, achieving varied outcomes.Arguments Provide the Actual Data
Arguments are the actual values passed to a function during execution. When you call a function, you provide arguments that replace the corresponding parameters. The function then uses these arguments to carry out its operations. For example:
Loading...
In this case,
Loading...
andLoading...
are arguments that replace parametersLoading...
andLoading...
, allowing the function to calculate their product.Matching Parameters and Arguments
One fundamental rule in JavaScript is that the number of arguments provided when calling a function should match the number of parameters the function expects. If there's a mismatch, you might encounter unexpected behavior or errors. This can occur in two ways:
-
Fewer Arguments Than Parameters: If you call a function with fewer arguments than it has parameters, the unspecified parameters become
Loading...
, which can lead to unexpected results orLoading...
(Not a Number) errors.Loading...
-
More Arguments Than Parameters: When a function receives more arguments than it has parameters, the extra arguments are ignored.
Loading...
Handling Mismatches with Default Parameters
To address scenarios with fewer arguments, JavaScript allows you to set default values for parameters. Default parameters ensure a function has a valid value, even when an argument is missing. This feature helps maintain robustness and avoid unexpected errors.
Loading...
In this example, default parameters avoid
Loading...
values, ensuring consistent behavior.Rest Parameters for Flexible Function Signatures
Sometimes, you might want a function that can accept a variable number of arguments. JavaScript provides rest parameters for this purpose. Using the
Loading...
syntax, you can create a function that accepts any number of arguments and processes them as an array. This technique allows for more flexible function signatures and can be helpful in various scenarios.Loading...
In this example, the
Loading...
function uses a rest parameter, allowing it to handle any number of arguments and calculate their total.The relationship between parameters and arguments is central to JavaScript functions. Parameters set the expectations for a function's input, while arguments provide the actual data when the function is called. Understanding this relationship is crucial for writing flexible, reusable code. It involves matching the number of parameters and arguments, using default parameters to avoid errors, and leveraging rest parameters for more flexible function signatures. You can write more robust and adaptable JavaScript code by mastering these concepts.
Practice Time
Here are some code snippets where you can fill in the missing parts to understand and practice JavaScript functions, parameters, and arguments.
Task 1: Function with Two Parameters
Define a function called
Loading...
that accepts two parameters,Loading...
andLoading...
, and returns their difference.Loading...
Task 2: Function with Default Parameters
Create a function called
Loading...
that takes a parameterLoading...
with a default value ofLoading...
. The function should return a greeting message using theLoading...
parameter.Loading...
Task 3: Function with Rest Parameters
Write a function called
Loading...
that accepts a variable number of arguments and returns their average.Loading...
Task 4: Function with Positional Arguments
Create a function called
Loading...
that takes three parameters:Loading...
,Loading...
, andLoading...
. The function should return the full name as a single string.Loading...
Task 5: Function with Parameter Validation
Write a function called
Loading...
that divides two numbers,Loading...
andLoading...
, but validates the parameters to ensure they are numbers andLoading...
is not zero. If these conditions are not met, throw an error.Loading...
These code snippets provide a range of exercises to help you practice defining functions, using parameters, passing arguments, and implementing validations. Fill in the blanks with appropriate values, and test your solutions to ensure they work as expected.
Happy Coding!!!!
** Book Recommendation: Eloquent JavaScript
Remember, if you get stuck, don't be afraid to look up solutions or ask for help. The key to learning programming is persistence! Ask for help - Mentorship
Join Our Discord Community Unleash your potential, join a vibrant community of like-minded learners, and let's shape the future of programming together. Click here to join us on Discord.
For Consulting and Mentorship, feel free to contact slavo.io