Understanding JavaScript Variables: A Comprehensive Guide
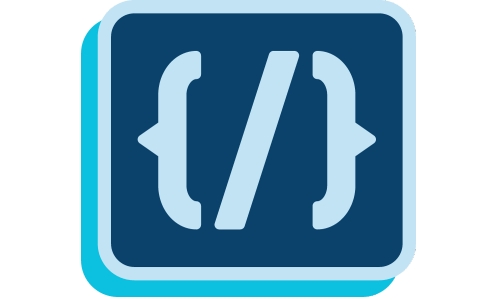
JavaScript, the cornerstone of modern web development, utilizes variables as a fundamental concept to store and manage data within programs. JavaScript variables can hold primitive data types like strings, numbers, and booleans or more complex structures like objects and arrays. This article will explore the reasons behind using variables, how to declare them, and the differences between
Loading...
,Loading...
, andLoading...
.** Book Recommendation: Eloquent JavaScript
Why We Need Variables
Variables act as containers for storing data values. They play a crucial role for several reasons:
- Memory Storage: Variables hold data in memory, enabling the retention, manipulation, and retrieval of values within the code.
- Code Reusability: Once a variable is defined, it can be used multiple times throughout the codebase, promoting reusability and maintainability.
- Dynamic Interaction: Variables facilitate dynamic user interactions on web pages by storing and manipulating values received from user inputs.
- Enhanced Readability: They allow developers to use descriptive names representing data, making the code more readable and understandable.
Declaring JavaScript Variables
Declaring a variable is like creating a box to store a value. In JavaScript, you can declare a variable using
Loading...
,Loading...
, orLoading...
, followed by the variable name, e.g.,Loading...
.Here’s a brief syntax illustration:
Loading...
Difference Between var, let, and const
1. var
Loading...
was the standard way to declare variables before ES6. It is function-scoped, meaning the variable's existence is limited to the function it was declared in. If declared outside any function, it becomes globally scoped. The main drawbacks are hoisting (where variable declarations are moved to the top of their enclosing scope during the execution phase) and being prone to redeclaration errors.Loading...
2. let
Introduced in ES6,
Loading...
allows block-scoped variable declaration, providing more control over variable's visibility. It resolves hoisting and global object property creation issues faced withLoading...
. UnlikeLoading...
, aLoading...
variable cannot be redeclared in the same scope.Loading...
3. const
Also introduced in ES6,
Loading...
is used to declare variables whose values should not be reassigned after their initial assignment, creating immutable variables. LikeLoading...
,Loading...
is block-scoped.Loading...
Recommendations
- Prefer using
Loading...
andLoading...
overLoading...
for the variable declaration to avoid hoisting and global object property issues and to make your code more readable and maintainable. - Use
Loading...
by default until you need to reassign the variable. At this point, useLoading...
. - Avoid using
Loading...
unless you have to support Internet Explorer or are working on legacy codebases.
Conclusion
JavaScript variables are indispensable for writing dynamic and efficient code. Understanding how and when to use
Loading...
,Loading...
, andLoading...
is crucial for managing data properly and writing more robust, error-free programs. By preferringLoading...
andLoading...
overLoading...
and adhering to good coding practices, developers can enhance code reliability and maintainability, ensuring smoother development experiences and more secure, high-performing applications.Below are a few simple exercises for practicing the declaration of variables using
Loading...
,Loading...
, andLoading...
in JavaScript. Try to complete them without looking at the solutions:Exercise 1: Basic Variable Declaration
- Declare a variable using
Loading...
and assign it a string value of your name. - Declare a variable using
Loading...
and assign it a number value representing your age. - Declare a variable using
Loading...
and assign it a boolean value representing whether you are a student.
Exercise 2: Redeclaring and Reassigning Variables
- Declare a variable using
Loading...
with the nameLoading...
and assign it the value of 'Paris'. - To redeclare the
Loading...
variable in the same block scope withLoading...
. - Reassign the
Loading...
variable to 'London'.
Exercise 3: Scoping
- Declare a
Loading...
variable inside a for loop and print its value outside it. - Declare a
Loading...
variable inside a for loop and try to print its value outside the loop. - Declare a
Loading...
variable inside an if block and try to print its value outside the block.
** Book Recommendation:
Remember, if you get stuck, don't be afraid to look up solutions or ask for help. The key to learning programming is persistence! Ask for help - Mentorship
Join Our Discord Community Unleash your potential, join a vibrant community of like-minded learners, and let's shape the future of programming together. Click here to join us on Discord.
For Consulting and Mentorship, feel free to contact slavo.io